HillShade
Function Description: Creates a hillshade based on the surface raster by considering the angle of the light source and shadows.
How It Works
The Hillshade tool generates a simulated illumination of the surface by determining the illumination value for each cell in the raster. This is achieved by setting the position of the light source and calculating the illumination values for each cell relative to its neighboring cells. It can significantly enhance the visual representation of the surface for analysis or graphical display, especially when using transparency.
By default, the shadows and light are associated with grayscale shading in integer values ranging from 0 to 255 (from black to white).
Hillshade Parameters
The primary factor in creating a hillshade map for any given location is the position of the sun in the sky.
Azimuth
The azimuth refers to the angular direction of the sun, measured clockwise in degrees from true north, within a range of 0 to 360 degrees. An azimuth of 90 degrees corresponds to the east. The default azimuth is 315 degrees (northwest).
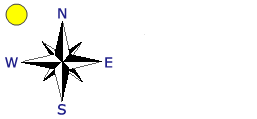
The default sun azimuth (direction) for hillshade is 315 degrees
Altitude
The altitude refers to the angle or slope of the light source above the horizon. The altitude is measured in degrees, ranging from 0 (on the horizon) to 90 (directly overhead). The default value is 45 degrees.
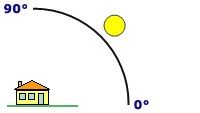
The default sun altitude for hillshade is 45 degrees
Hillshade Example
The following hillshade example uses an azimuth of 315 degrees and an altitude of 45 degrees.
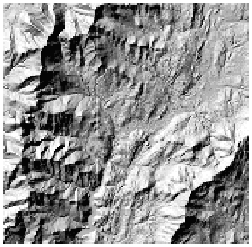
Output example of Hillshade
Hillshade Calculation
The algorithm used to calculate hillshade values is as follows:
Hillshade = 255.0 * ((cos(Zenith_rad) * cos(Slope_rad)) + (sin(Zenith_rad) * sin(Slope_rad) * cos(Azimuth_rad - Aspect_rad)))
Note that if the calculation of the hillshade value is less than 0, the output cell value will be equal to 0.
Compute the illumination angle
The altitude of the illumination source is specified in degrees above horizontal. However, the formula for calculating the hillshade value requires that the angle be represented in radians and be the deflection from the vertical. The direction straight up from the surface (directly overhead) is labeled the zenith. The zenith angle is measured from the zenith point to the direction of the illumination source and is the 90 degrees complement to the altitude. To calculate the illumination angle, first convert the altitude angle to the zenith angle. Next, convert the angle to radians.
Change altitude to zenith angle:
Zenith_deg = 90.0 - Altitude
Convert to radians:
Zenith_rad = Zenith_deg * pi / 180.0
Compute the illumination direction
The direction of the illumination source, azimuth, is specified in degrees. The hillshade formula requires this angle to be in units of radians. First, change the azimuth angle from its geographic unit (compass direction) to a mathematic unit (right angle). Next, convert the azimuth angle to radians.
Change azimuth angle measure:
Azimuth_math = 360.0 - Azimuth + 90.0
Note that if Azimuth_math >= 360.0, the following is true:
Azimuth_math = Azimuth_math - 360.0
Convert to radians:
Azimuth_rad = Azimuth_math * pi / 180.0
Compute slope and aspect
A moving 3-by-3 window moves to each cell in the input raster, and for each cell in the center of the window, an aspect and slope value are calculated using an algorithm that incorporates the values of the cell's eight neighbors. The cells are identified as letters a to i, with e representing the cell for which the aspect is being calculated.
The rate of change in the x direction for cell e is calculated with the following algorithm:
[dz/dx] = ((c + 2f + i) - (a + 2d + g)) / (8 * cellsize)
The rate of change in the y direction for cell e is calculated with the following algorithm:
[dz/dy] = ((g + 2h + i) - (a + 2b + c)) / (8 * cellsize)
The steepest downhill descent from each cell in the surface is the slope. The algorithm for calculating the slope in radians, incorporating the z-factor, is as follows:
Slope_rad = ATAN (z_factor * √ ([dz/dx]2 + [dz/dy]2))
The direction the steepest downslope direction is facing is the aspect. Aspect in radians is defined in the range of 0 to 2pi, with 0 toward east. The aspect is determined under the rules in the following algorithm:
If [dz/dx] is non-zero:
Aspect_rad = atan2 ([dz/dy], -[dz/dx])
if Aspect_rad < 0 then
Aspect_rad = 2 * pi + Aspect_rad
If [dz/dx] is zero:
if [dz/dy] > 0 then
Aspect_rad = pi / 2
else if [dz/dy] < 0 then
Aspect_rad = 2 * pi - pi / 2
else
Aspect_rad = Aspect_rad
Hillshade calculation example
In this example, the hillshade value of the center cell of the moving window is calculated.
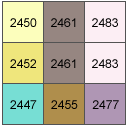
Hillshade calculation example
The cell size is 5 units. The default Altitude of 45 degrees and Azimuth of 315 degrees will be used.
Illumination angle
The calculation for zenith angle from equation 2 is as follows:
Zenith_deg = 90.0 - Altitude
= 90.0 - 45.0
= 45.0
And converted to radians from equation 3 is as follows:
Zenith_rad = Zenith_deg * pi / 180.0
= 45.0 * 3.1415926536 / 180.0
= 0.7853981634
Illumination direction
The calculation for converting the azimuth angle from geographic to mathematic angle with equation 4 is as follows:
Azimuth_math = 360.0 - Azimuth + 90.0
= 360.0 - 315.0 + 90.0
= 135.0
Converting azimuth angle to radians with equation 6 is as follows:
Azimuth_rad = Azimuth_math * pi / 180.0
= 135.0 * 3.1415926536 / 180
= 2.3561944902
Slope and aspect
The calculation for the rate of change in the x direction for center cell e is as follows:
[dz/dx] = ((c + 2f + i) - (a + 2d + g)) / (8 * cellsize)
= ((2483 + 4966 + 2477) - (2450 + 4904 + 2447)) / (8 * 5)
= (9926 - 9801) / 40
= 3.125
The calculation for the rate of change in the y direction for center cell e is as follows:
[dz/dy] = ((g + 2h + i) - (a + 2b + c)) / (8 * cellsize) = (2447 + 4910 + 2477) - (2450 + 4922 + 2483) / (8 * 5)
= (9834 - 9855) / 40
= -0.525
The calculation for the slope angle is as follows:
Slope_rad = ATAN ( z_factor * √ ([dz/dx]2 + [dz/dy]2))
= atan(1 * sqrt((3.125 * 3.125) + (-0.525 * -0.525)))
= atan(1 * sqrt(10.04125 + 0.275625))
= atan(1 * 3.1687931457)
= 1.2651101670
The calculation for the Aspect_rad angle from rule 10 is as follows (since dz/dx is nonzero in this example):
Aspect_rad = atan2 ([dz/dy], -[dz/dx])
= atan2(-0.525, -3.125)
= -2.9751469600
Since this value is less than 0, this part of the rule applies:
Aspect_rad = 2 * pi + Aspect_rad
= 2 * 3.1415926536 + -2.9751469600
= 3.3080383471
Hillshade
The final calculation for the hillshade is as follows:
Hillshade = 255.0 * ((cos(Zenith_rad) * cos(Slope_rad)) + (sin(Zenith_rad) * sin(Slope_rad) * cos(Azimuth_rad - Aspect_rad)))
= 255.0 * ((cos(0.7857142857) * cos(1.26511)) + (sin(0.7857142857) * sin(1.26511) * cos(2.3571428571 - 3.310567)))
= 153.82
Since the output raster is of integer type, the shade value for center cell e = 154.
Data Description
Input and Output Relationship
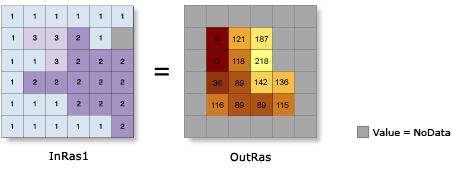
Hillshade Input and Output Relationship
- The Hillshade tool creates a hillshade raster based on a given raster, treating the light source as if it is at an infinite distance.
- The integer values of the hillshade raster range from 0 to 255.
- Shadow analysis is performed by accounting for the influence of the local horizon on each cell. Cells in shadow are assigned a value of zero.
Steps
- Go to Toolbox -> Raster Tools -> Surface Analysis -> Hillshade tool, and double-click to open the tool dialog.
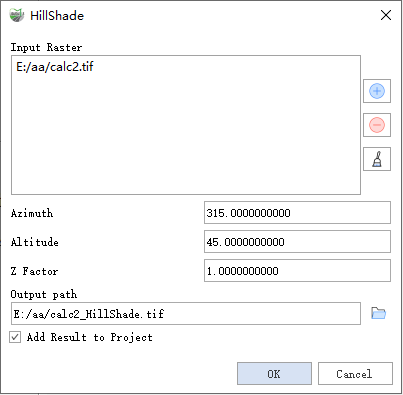
HillShade
Parameter Description:
- Input Raster: The input surface raster.
- Azimuth: The azimuth of the light source, expressed as a positive degree value between 0 and 360. It is measured clockwise from true north, with a default value of 315 degrees.
- Altitude: The altitude angle of the light source above the horizon, expressed as a positive degree value. An altitude of 0 degrees represents the horizon, while 90 degrees represents directly overhead. The default value is 45 degrees.
- Z Factor: The number of ground units (x, y) per surface z unit. When the z units differ from the x, y units of the input surface, the Z factor adjusts the measurement units of the z values. The Z factor is multiplied by the z values of the input surface during calculation. If the x, y units and z units use the same measurement units, the Z factor is 1 (default setting). If they use different units, the Z factor must be set appropriately to ensure correct results. For example, if the z units are in feet and the x, y units are in meters, use a Z factor of 0.3048 to convert feet to meters (1 foot = 0.3048 meters).
- Output path: The output hillshade raster. The hillshade raster values range from 0 to 255.
- Add Result to Project: If checked, a layer for the result data will be automatically created and added to the project.